Temperature Converter App : Android Basic App
1. Start Android Studio
First, open Android Studio. You should see this window. Go ahead and click "Start a new Android Studio Project". An Android Studio Project typically means the code and files for one Android Application.
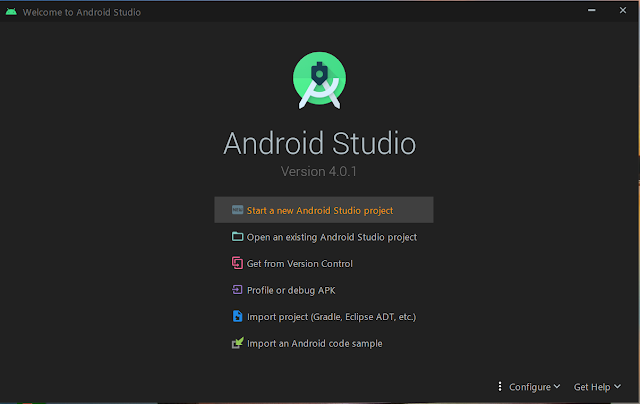
2. Choose your Project
On the next page you will be presented with several device platform tabs, each with different templates for adding an Activity.
From the "Phone and Tablet" tab choose "Empty Activity," and click "Next" again.
3. Configure Android Project
Configure your project
For this app use this configuration:
Name: TempConverter
Package: com.example.android.diceroller
Project location: < Your choice of where to save this project on your computer >
Language: Java
Minimum API: leave the default values
4. Now Design the App
Design your app using Android Studio.
Design Code :
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/text_heading"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:padding="8sp"
android:text="@string/fahrenheit_to_celcius"
android:textColor="@android:color/black"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="298dp"
android:layout_height="240dp"
android:layout_marginTop="32dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/text_heading"
app:srcCompat="@drawable/temp" />
<EditText
android:id="@+id/edit_far"
android:layout_width="160dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="52dp"
android:ems="10"
android:hint="@string/enter_temp_in_f"
android:inputType="numberDecimal"
app:layout_constraintEnd_toStartOf="@+id/btn_tocel"
app:layout_constraintHorizontal_bias="0.278"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<Button
android:id="@+id/btn_tocel"
android:layout_width="140dp"
android:layout_height="wrap_content"
android:layout_marginEnd="16dp"
android:backgroundTint="@color/red"
android:text="@string/to_celcius"
android:textColor="@android:color/background_light"
app:layout_constraintBottom_toBottomOf="@+id/edit_far"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="@+id/edit_far"
app:layout_constraintVertical_bias="0.0" />
<EditText
android:id="@+id/edit_cel"
android:layout_width="160dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="28dp"
android:layout_marginEnd="12dp"
android:ems="10"
android:hint="@string/entertemp_in_c"
android:inputType="numberDecimal"
app:layout_constraintEnd_toStartOf="@+id/btn_tofar"
app:layout_constraintHorizontal_bias="0.338"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/edit_far" />
<Button
android:id="@+id/btn_tofar"
android:layout_width="140dp"
android:layout_height="wrap_content"
android:layout_marginEnd="16dp"
android:backgroundTint="@color/blue"
android:text="@string/to_fahrenheit"
android:textColor="@android:color/background_light"
app:layout_constraintBottom_toBottomOf="@+id/edit_cel"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="@+id/edit_cel"
app:layout_constraintVertical_bias="0.0" />
</androidx.constraintlayout.widget.ConstraintLayout>
4. Adding Some Resources:
Addning required Strings to strings.xml:
<resources>
<string name="app_name">TempConverter</string>
<string name="action_settings">Settings</string>
<!-- Strings used -->
<string name="fahrenheit_to_celcius">Celcius ⇆ Fahrenheit</string>
<string name="to_celcius">To °Celcius</string>
<string name="enter_temp_in_f">Enter Temp in °F</string>
<string name="entertemp_in_c">EnterTemp in °C</string>
<string name="to_fahrenheit">To °Fahrenheit</string>
</resources>
Adding Colors to colors.xml:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#fb8c00</color>
<color name="colorPrimaryDark">#c25e00</color>
<color name="colorAccent">#ffc947</color>
<color name="blue">#039BE5</color>
<color name="red">#E53935</color>
</resources>
Generated Layout will be like this:
5. Adding Java Code:
MainActivity.java
package com.example.tempconverter;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
//Declaring Variables
EditText editFar,editCel;
Button btnToFar,btnToCel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Initilizing variables"
editFar = findViewById(R.id.edit_far);
editCel = findViewById(R.id.edit_cel);
btnToCel = findViewById(R.id.btn_tocel);
btnToFar = findViewById(R.id.btn_tofar);
//To convert temperature from fahrenheit to celsius
btnToCel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
toCel();
}
});
//To convert temperature from celsius to fahrenheit
btnToFar.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
toFar();
}
});
}
//function to convert temp in fahrenheit to celsius
private void toFar() {
Double tempInCel = Double.parseDouble(editCel.getText().toString());
Double tempInFar = (tempInCel*9/5)+32;
Toast.makeText(this,tempInFar.toString()+" ℉",Toast.LENGTH_LONG).show();
}
// function to convert temp to celsius
private void toCel(){
Double tempInFar = Double.parseDouble(editFar.getText().toString());
Double tempInCel = (tempInFar - 32) * 5/9;
Toast.makeText(this,tempInCel.toString()+" ℃",Toast.LENGTH_LONG).show();
}
}
Yay!! Our App is ready!!
1.Converting Fahrenheit to Celcius
2.Converting Celsius to Fahrenheit
Comments
Post a Comment